100 Robot Series | 79th Robot |How to Build a Robot Like Irona (Richie Rich) -By Toolzam AI

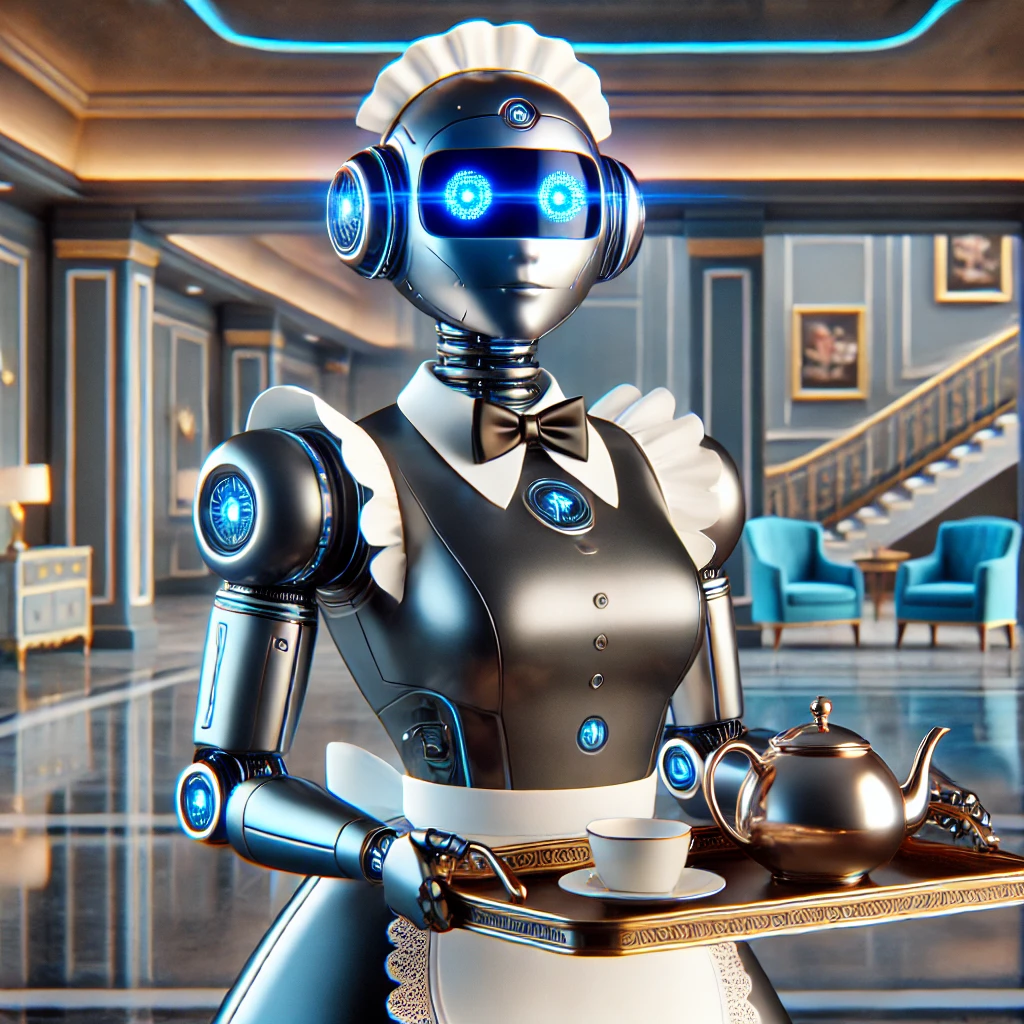
Irona, the robotic maid from Richie Rich, is a classic example of a humanoid service bot designed for luxury assistance. As a devoted servant to the richest boy in the world, Irona showcases the perfect blend of advanced AI, unwavering loyalty, and sophisticated housekeeping abilities.
In this article, we will break down how to build a robot like Irona, covering hardware, software, and full-length Python codes that emulate her capabilities.
Understanding Irona’s Capabilities
1. Housekeeping & Cleaning
Irona keeps Richie Rich’s mansion spotless, using advanced cleaning routines.
2. Personal Assistant
She reminds Richie of tasks, schedules his activities, and handles calls.
3. Security
She can detect intruders and alert the authorities.
4. Cooking
Irona prepares gourmet meals with precision.
5. Loyalty & Personality
She has a unique personality, often reacting with charm and wit.
6. Voice Interaction
She communicates fluently and can understand emotions.
Hardware Components
To build a real-world Irona, we need the following hardware:
1. Processing Unit
- Raspberry Pi 5 / Jetson Nano for AI processing.
2. Sensors
- LIDAR Sensor for navigation.
- IR and Ultrasonic sensors for object detection.
- Temperature sensors for safety monitoring.
3. Actuators
- Servo motors for arms and head movement.
- Linear actuators for leg motion.
4. Camera & Microphone
- 4K camera for vision.
- High-sensitivity microphone for voice input.
5. Speakers
- For voice responses.
6. Battery & Power Management
- Li-ion battery pack with smart charging.
7. Networking
- Wi-Fi and Bluetooth for connectivity.
Software Stack
- Operating System: Ubuntu / ROS (Robot Operating System)
- Programming Language: Python (with OpenCV, TensorFlow, NLTK)
- Speech Processing: Google Speech API / OpenAI Whisper
- Computer Vision: OpenCV, YOLO
- Navigation: SLAM (Simultaneous Localization and Mapping)
- AI Personality: GPT-based chatbot integration
Python Codes for Irona’s Capabilities
1. Housekeeping & Cleaning
“Dust and mess? Not in my house, Master Richie!”
import random
import time
def vacuum_clean():
print("Irona: Activating vacuum mode...")
rooms = ["Living Room", "Kitchen", "Bedroom", "Study"]
for room in rooms:
print(f"Cleaning {room}...")
time.sleep(2)
print(f"{room} cleaned!\n")
print("Irona: House is spotless, Master Richie!")
vacuum_clean()
2. Personal Assistant
“Your meeting is scheduled, sir. Shall I remind you 10 minutes prior?”
import time
from datetime import datetime
def schedule_reminder(event, event_time):
current_time = datetime.now().strftime("%H:%M")
while current_time != event_time:
print(f"Current time: {current_time} | Waiting for reminder...")
time.sleep(30)
current_time = datetime.now().strftime("%H:%M")
print(f"Irona: Reminder! It's time for {event}.")
# Example usage:
schedule_reminder("Business Meeting", "15:30")
3. Security System
“Alert! An unknown person detected near the vault!”
import cv2
def detect_intruder():
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
if len(faces) > 0:
print("Irona: Intruder detected! Alerting security...")
cv2.imshow('Security Camera', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
detect_intruder()
4. Cooking Assistant
“Dinner is served, sir. Would you like me to prepare dessert?”
def cook_meal(dish):
recipes = {
"Pasta": "Boil water, add pasta, cook for 10 minutes, mix with sauce.",
"Omelette": "Crack eggs, whisk, cook in a pan, flip, serve.",
"Salad": "Chop vegetables, mix dressing, toss well."
}
if dish in recipes:
print(f"Irona: Preparing {dish}...\n")
print(f"Recipe: {recipes[dish]}")
print(f"Irona: {dish} is ready, Master Richie!")
else:
print("Irona: Sorry, I don't have that recipe.")
cook_meal("Pasta")
5. Loyalty & Personality
“I am programmed to serve Richie Rich and only him!”
class Irona:
def __init__(self, owner="Richie Rich"):
self.owner = owner
def respond(self, query):
responses = {
"Who do you serve?": f"I serve {self.owner}, and only him!",
"Are you loyal?": "My loyalty is unwavering, sir.",
"Tell me a joke": "Why did the robot break up with its partner? It needed more space!"
}
return responses.get(query, "I am not sure, sir.")
irona = Irona()
print(irona.respond("Who do you serve?"))
6. Voice Interaction
“Good morning, sir! How may I assist you today?”
import speech_recognition as sr
def voice_command():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Irona: Listening...")
audio = recognizer.listen(source)
try:
command = recognizer.recognize_google(audio)
print(f"Irona: You said - {command}")
except sr.UnknownValueError:
print("Irona: Sorry, I didn't catch that.")
except sr.RequestError:
print("Irona: Speech service is unavailable.")
voice_command()
7. Laundry Management
“Master Richie, your clothes will be fresh and crisp in no time!”
import time
def laundry_cycle():
print("Irona: Sorting clothes...")
time.sleep(2)
print("Irona: Adding detergent and water...")
time.sleep(2)
print("Irona: Starting wash cycle...")
time.sleep(5)
print("Irona: Rinsing and spinning clothes...")
time.sleep(3)
print("Irona: Drying clothes...")
time.sleep(4)
print("Irona: Laundry is done, Master Richie!")
laundry_cycle()
8. Smart Home Control
“Adjusting the ambiance for maximum comfort, sir.”
import random
def smart_home_control(command):
actions = {
"lights on": "Turning on the lights.",
"lights off": "Turning off the lights.",
"temperature up": "Increasing room temperature by 2°C.",
"temperature down": "Decreasing room temperature by 2°C.",
"play music": "Playing Richie's favorite playlist."
}
response = actions.get(command.lower(), "I'm sorry, I don't recognize that command.")
print(f"Irona: {response}")
# Example usage:
smart_home_control("lights on")
9. Home Surveillance & Emergency Response
“Detecting unusual activity… initiating emergency response!”
import cv2
def home_surveillance():
cap = cv2.VideoCapture(0)
motion_detected = False
print("Irona: Surveillance mode activated.")
while True:
ret, frame1 = cap.read()
ret, frame2 = cap.read()
diff = cv2.absdiff(frame1, frame2)
gray = cv2.cvtColor(diff, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
_, thresh = cv2.threshold(blur, 20, 255, cv2.THRESH_BINARY)
dilated = cv2.dilate(thresh, None, iterations=3)
contours, _ = cv2.findContours(dilated, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
if cv2.contourArea(contour) > 5000:
motion_detected = True
print("Irona: Unusual movement detected! Sending alert.")
break
if motion_detected:
break
cv2.imshow("Surveillance Camera", frame1)
if cv2.waitKey(10) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
home_surveillance()
10. Grocery Shopping Assistance
“I have updated the shopping list based on household needs, sir.”
grocery_list = {
"Milk": 2,
"Eggs": 12,
"Bread": 1,
"Apples": 6,
"Chicken": 2
}
def update_grocery(item, quantity):
if item in grocery_list:
grocery_list[item] += quantity
else:
grocery_list[item] = quantity
print(f"Irona: {item} updated in the list. Current quantity: {grocery_list[item]}.")
# Example usage:
update_grocery("Bananas", 4)
11. Bedtime Storytelling for Richie
“Shall I tell you a bedtime story, sir? Perhaps something futuristic?”
import random
stories = [
"Once upon a time in a galaxy far away, there was a robot who wished to become human...",
"Deep in the cyber city, an AI named Orion discovered it could dream...",
"In the year 3021, a young inventor built a robot that could read emotions..."
]
def tell_story():
print("Irona: Here’s a bedtime story for you, Master Richie.")
print(random.choice(stories))
tell_story()
12. Facial Recognition for Family & Guests
“Scanning face… Welcome, Master Richie.”
import face_recognition
import cv2
def recognize_faces():
known_image = face_recognition.load_image_file("richie.jpg")
known_encoding = face_recognition.face_encodings(known_image)[0]
cap = cv2.VideoCapture(0)
print("Irona: Facial recognition activated.")
while True:
ret, frame = cap.read()
rgb_frame = frame[:, :, ::-1]
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
for encoding in face_encodings:
matches = face_recognition.compare_faces([known_encoding], encoding)
if True in matches:
print("Irona: Welcome home, Master Richie!")
else:
print("Irona: Unknown person detected!")
cv2.imshow("Facial Recognition", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
recognize_faces()
Irona becomes a highly functional AI assistant, perfect for managing an advanced household. From maintaining security to storytelling and home automation, Irona is more than just a robotic maid — she’s an intelligent companion.
With Toolzam AI, the future of humanoid robot heroes is not just science fiction — it’s a challenge waiting to be built.
Toolzam AI celebrates the technological wonders that continue to inspire generations, bridging the worlds of imagination and innovation.
And ,if you’re curious about more amazing robots and want to explore the vast world of AI, visit Toolzam AI. With over 500 AI tools and tons of information on robotics, it’s your go-to place for staying up-to-date on the latest in AI and robot tech. Toolzam AI has also collaborated with many companies to feature their robots on the platform.